Creating a Basic API with Rails using Active Model Serializer (AMS) in less than 5 minutes
19 April 2019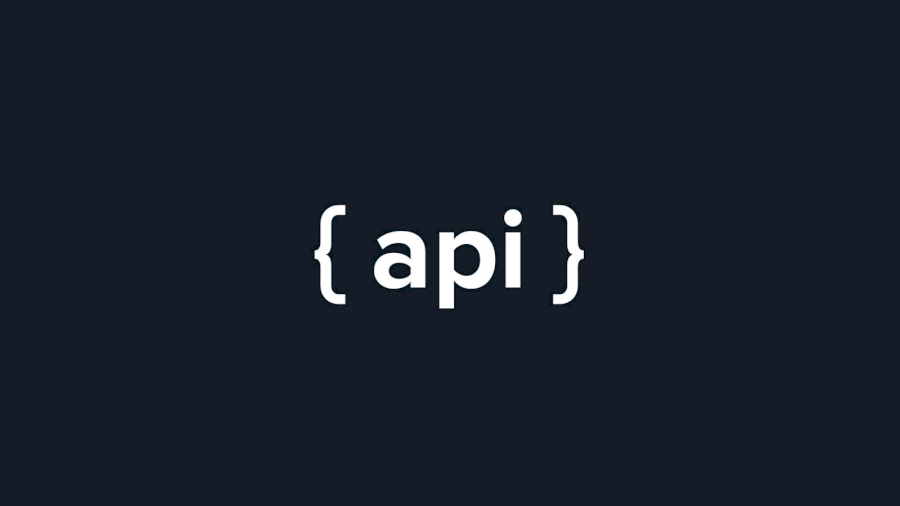
Do you know Rails? Active Record? Can you have a Rails Web App up and running real quick but struggle to do a Web API? This article is for you!
Let me introduce you to a tool called Active Model Serializer (AMS):
Active Model Serializer is a gem that allow you to create complex APIs using the same active record associations that you’re already used to. Let’s dive in!
First, go to your gem file and paste: gem 'active_model_serialiers'
then go in you console and type bundle
Now let’s say you have a Web App where users can follow courses and that your course SQLite schema looks like this:
create_table "courses", force: :cascade do |t|
t.string "title" t.text "content" t.integer "user_id"
t.datetime "created_at", null: false
t.datetime "updated_at", null: false
end
Assuming you want to expose a course trough your API you will have to go through these steps:
- - Create a
CourseSerializer.rb
file (You can do that using a special rails migration that comes with the AMS gem. - - Specify which fields you want to expose through API in you serializer file
- - Modify your controller to render
json
…and Voila!
Step 1
Go to your console and type rails g serializer Course
. This will automatically create a serializers directory that will contain you serializer file. Go in the file and should look like this:
Step 2
In the attributes section, add the attribute that you would like to see rendered in the json later. For example you may want to add the content of the course in the attributes and will be shown in you json. It’s that simple!
At this point, you may be asking yourself about how you would include a field containing an associated model (the user for example). You can simply add the association in the serializer file and all will be taken care of. You will have to create a serializer file for the user too using the same migration. For example, a course belongs to a user and I want to display the user information nested inside the course. First I will create the user serializer, add the attributes I want to see from the user in it, then add the association has_many :courses
beneath the attributes section. Next, I go to the course serializer and add belongs_to :users
. That’s it!
Last Step
The Last Step is to go to your controller, let say we want to list all courses and display them as json. I will just go to my index action and add the following: render json: @courses , status: 200
. The whole action will look like this:
def index @courses=Course.all render json: @courses, status: 200 end
Go in the index routes and you will see all the courses displayed before your eyes as JSON.
There is much more available with the active_model_serializers
gem so you can look at his public repo on GitHub.
Thanks for reading!