How to send Transactions in Filecoin using Ethers.js
16 November 2023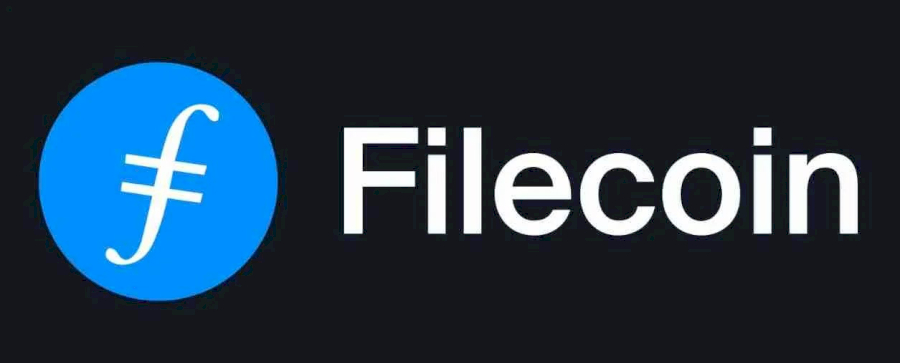
Filecoin is a decentralized storage network that enables users to store and retrieve data using a blockchain-based market. If you're interested in interacting with Filecoin using JavaScript, specifically with the Ethers.js library, you can follow the general steps outlined below.
As legacy transactions are not allowed in Filecoin chain, sending transaction without data as it is usual in EVM chains can add extra troubles in FVM, which may lead to excees problems in your project.
You might see an error message like 'legacy transaction is not supported ' so let's solve it.
So in this post we will explain everything with examples. Our next post will be about FilForwarder to be able to send funds from 0x address to f0, f1, f2, f3 native Filecoin addresses
Install Ethers.js:
Ensure that you have Node.js and npm installed on your system. You can then install Ethers.js using the following command:
npm install ethers
Begin with the Code
const { BigNumber } = require('ethers'); const ethers = require('ethers'); const provider = new ethers.providers.JsonRpcProvider('RPC URL'); async function main() { const from = "FROM_ADDRESS"; const to = "TO_ADDRESS"; const value = 123000000000000n; const estimGas = Number(await provider.estimateGas({from: from, to: to, value: value})); feeData = await provider.getFeeData(); const nonce = await provider.getTransactionCount(from, 'latest'); // Example message for gas estimation const transaction = { type: 2, // remove in case of ETH and BNB, is used for chains where lecgacy transactions are not allowed to: to, nonce: nonce, value: value, gasLimit: estimGas,//5000000n, //2152567 (for FIL testnet) chainId: 314159, maxFeePerGas: BigNumber.from(feeData.maxFeePerGas), // is used with type 2 maxPriorityFeePerGas: BigNumber.from(feeData.maxPriorityFeePerGas), //1500000000n, is used with type 2 }; const privateKey = process.env.SIGNER; const wallet = new ethers.Wallet(privateKey, provider); const signedTransaction = await wallet.signTransaction(transaction); // // Send the signed transaction const transactionResponse = await provider.sendTransaction(signedTransaction); transactionResponse.wait(); // // return transactionResponse; console.log('Transaction Hash:', transactionResponse); } // Call the function to estimate gas main() .then(() => process.exit(0)) .catch((error) => { console.error(error); process.exit(1); });
You can also read our Related articles
How to send Transactions in Filecoin using web3js