How to send Transactions in Filecoin using web3js
08 October 2023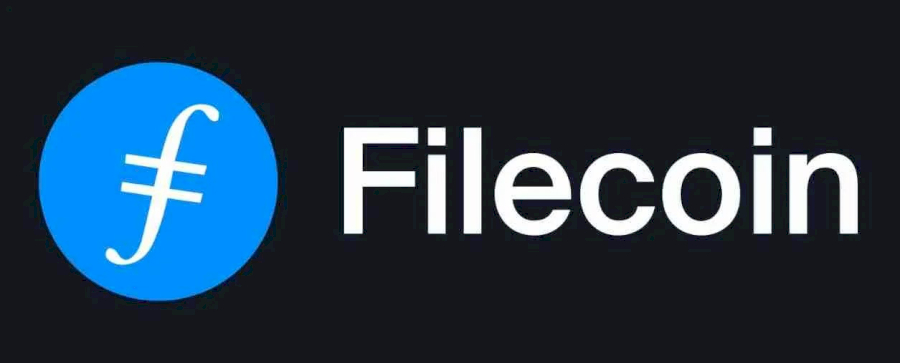
Filecoin is a decentralized storage network that enables users to store and retrieve data using a blockchain-based market. If you're interested in interacting with Filecoin using JavaScript, specifically with the Web3.js library, you can follow the general steps outlined below.
As legacy transactions are not allowed in Filecoin chain, sending transaction without data as it is usual in EVM chains can add extra troubles in FVM, which may lead to excees problems in your project.
You might see an error message like 'legacy transaction is not supported ' so let's solve it.
So in this post we will explain everything with examples. Our next post will be about FilForwarder to be able to send funds from 0x address to f0, f1, f2, f3 native Filecoin addresses
Install Web3.js:
Ensure that you have Node.js and npm installed on your system. You can then install Web3.js using the following command:
npm install web3
In your JS file
const fileCoinUrl:string = env.NODE_FILECOIN_HTTPS || 'https://filecoin.public-rpc.com'; export const web3Filecoin = new Web3(fileCoinUrl); const account = web3Filecoin.eth.accounts.privateKeyToAccount(env.PRIVATE_KEY) web3Filecoin.eth.accounts.wallet.add(account); const gasPrice = Number(await web3Filecoin.eth.getGasPrice()); // Get Gas Price const amountToWei =web3Filecoin.utils.toWei(amount,'ether'); // Covert Number to BN (WEI) const gasAmount = await web3Filecoin.eth.estimateGas({ from: account.address, to, value:amountToWei, }); // Estimate Gas Fee const transaction = { from:account.address, to, value: amountToWei, maxPriorityFeePerGas: 300000, gasLimit:gasAmount , } maxPriorityFeePerGas is used to define how much you are willing to pay to Miner, so in mainnet it is usually between 100000 to 300000. web3Filecoin.eth.accounts.signTransaction(transaction, env.PRIVATE_KEY).then(signedTx => { if(!signedTx){ console.log('Gas price is too high') return; } web3Filecoin.eth.sendSignedTransaction(signedTx.rawTransaction as string); })
You can also read our Related articles
How to send Transactions in Filecoin using Ethers.js