How to use FilForwarder with Web3 js For FileCoin network
16 November 2023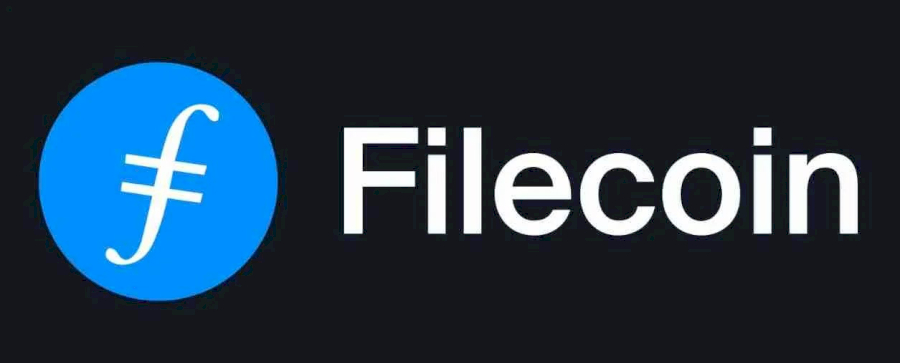
As Filecoin natively supports 0x (EVM) like addreses, it may be a problem to send funds from 0x address to f0 or any other native address. For that reason Filecoin is providing a contract FilForwarder which is solving this issue. FilForwarder address is always the same in all networks (mainnet, testnet).
In documentations of Filecoin or Lotus you will find the code which is used in hardhat, and this is not what you might need for your web project. So that is why we are covering web3.js part
So let's begin with code
npm install web3 npm install @zondax/izari-tools const { Address } = require('@zondax/izari-tools'); const Web3 = require('web3'); const providerUrl = "https://rpc.ankr.com/filecoin/"; const web3 = new Web3(providerUrl); async function forward(destination, amount) { const from = await web3.eth.accounts.privateKeyToAccount(env.PRIVATE_KEY); const destinationAddress = Address.fromString(destination); const wei = web3.utils.toWei(amount); // forwarderAddress is always the same in mainnet and testnet so you don't really have to change it const forwarderAddress = "0x2B3ef6906429b580b7b2080de5CA893BC282c225"; const contractABI = [ { "constant": true, "inputs": [ { "name": "destination", "type": "bytes" } ], "name": "forward", "outputs": [], "payable": true, "stateMutability": "payable", "type": "function" } ] // Create a contract instance const filForwarder = new web3.eth.Contract(contractABI,forwarderAddress); console.log(filForwarder.methods) const txData = filForwarder.methods.forward(destinationAddress.toBytes()).encodeABI(); const gasLimit = await filForwarder.methods.forward(destinationAddress.toBytes()).estimateGas({ from: from.address,value:wei }); const nonce = await web3.eth.getTransactionCount(from.address, 'latest'); const txParams = { to: forwarderAddress, data: txData, type:'0x2', // important for non legacy transactions, EIP1559 value: wei, // Send value in wei gas: gasLimit, // Replace with your desired gas limit }; web3.eth.accounts.signTransaction(txParams, privateKey) .then(signedTx => { // Send the signed transaction return web3.eth.sendSignedTransaction(signedTx.rawTransaction); }) .then(receipt => { console.log('Transaction Hash:', receipt.transactionHash); }) .catch(error => { console.error(error); }); }
You can also read our post